Here is the PHP code I use to redirect to a custom WordPress Admin Page when I log into WordPress as an Administrator.
Add this in functions.php or wherever else you add PHP code snippets (using WPCodeBox etc).
// redirect our Dashboard > Home page to a custom url
add_action('admin_init', 'c_admin_redirects');
function c_admin_redirects() {
if ( is_plugin_active('plausible-analytics/plausible-analytics.php') ) {
global $pagenow;
/* Redirect to Plausible Analytics */
if($pagenow == 'index.php' && !isset($_GET['page'])){
wp_redirect(admin_url("index.php?page=plausible-analytics-statistics", 'https'), 301);
exit;
}
}
}
Code language: PHP (php)
But the above PHP code isn’t going to be very useful if you don’t know how to change it to suit your needs and redirect to the page you need it to.
So let’s do that in this article today.
Let’s build up this function as we proceed through this article today and we’ll go through what each line of code is doing as we go along.
The Video Tutorial
Here is a video walkthrough of this tutorial.
Step 1: The admin_init hook VS login_redirect
Let’s start building up this function, starting with the first line – the hook…
// redirect our Dashboard > Home page to a custom url
add_action('admin_init', 'c_admin_redirects');
function c_admin_redirects() {
}
Code language: PHP (php)
So the first thing about this code is that it fires on the admin_init
WordPress hook.
The WordPress website explains this hook in the following way:
“Fires as an admin screen or script is being initialized.”
It also goes on to explain…
admin_init
is triggered before any other hook when a user accesses the admin area.
This is exactly why we want to use this hook and not another one.
This way, we can trigger the redirect before anything else happens on the page and files start downloading and the page begins to render.
Now, I want to point out that we are NOT using the login_redirect
WordPress filter and doing something like the below code where we redirect administrators to a custom page when they Log into WordPress.
add_filter( 'login_redirect', 'c_login_redirect', 10, 3 );
function c_login_redirect( $url, $request, $user ) {
if ( $user && is_object( $user ) && is_a( $user, 'WP_User' ) ) {
if ( $user->has_cap( 'administrator' ) ) {
$url = admin_url("index.php?page=plausible-analytics-statistics", 'https')
} else {
$url = home_url();
}
}
return $url;
}
Code language: PHP (php)
The reason?
Because the login_redirect
filter will only run ONCE – when a user with the user role of “administrator” initially logs into WordPress.
But I am always logged into WordPress – writing blog posts and tinkering *insert time wasting?*
So for me, redirecting on login would be meaningless as the redirect would hardly ever fire.
And that’s the reason I have opted to use the admin_init
hook over login_redirect
for the code.
Now, back to code and how it works…
On my website (where this code was taken from) I have a plugin installed named Plausible Analytics.
It’s an alternative to Google Analytics 4 but it has a small cost ($9 or something) so I want to put these analytics in front of me as much as possible during the trial period so I can decide if it’s worth paying for once the trial ends.
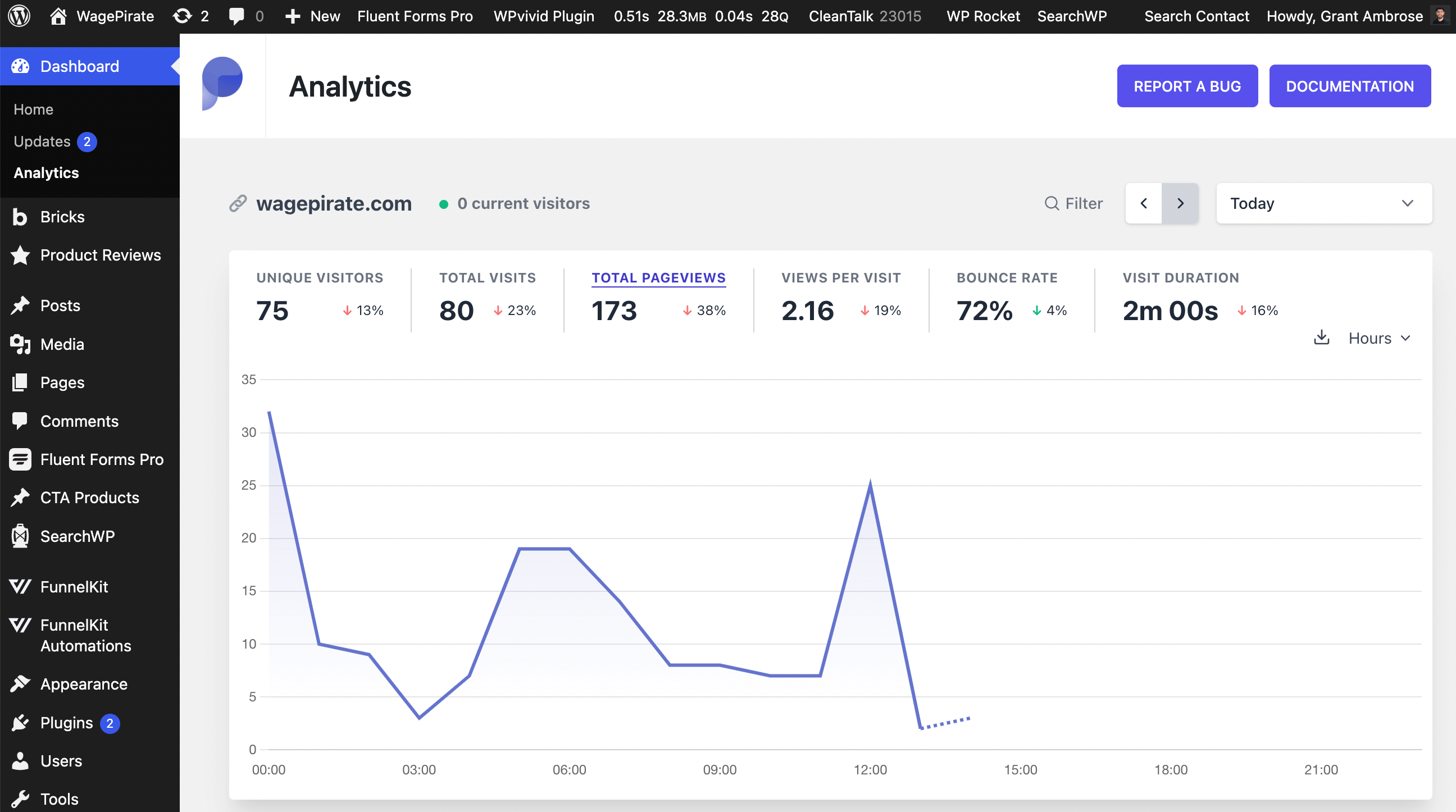
With that considered…
A link I always click on is the one below…
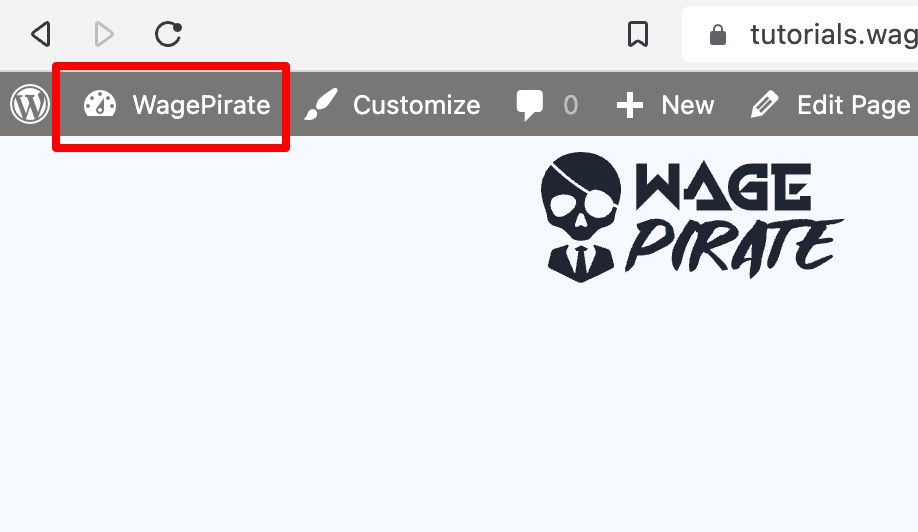
You probably know this – but clicking this takes you from the front-end of my website to my dashboard and vice versa.
I’m always doing this while managing my website.
Now – when you click that link from the front-end, you normally land on the page Dashboard > Home (1) and the URL is https://<yourdomain.com>/wp-admin/
(2).
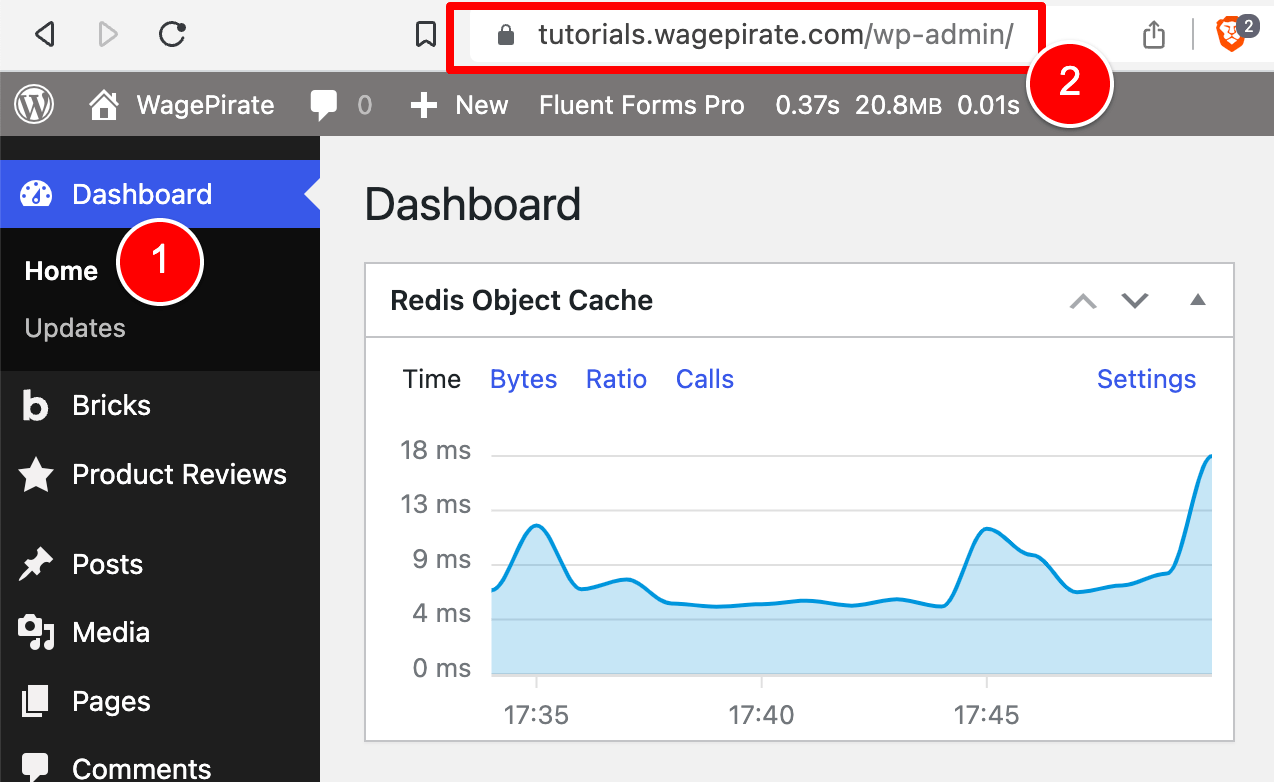
This page has never been useful for me and I always find myself loading it and then jumping straight to another page without even taking a second to glance at what is on this screen.
So combining the fact that I want to see my Plausible Analytics more with the fact that I never use this Dashboard > Home page…
It makes sense for me to set up a redirect for this URL so that whenever I try to access this Dashboard > Home page (index.php) I send myself to the analytics page for the Plausible Analytics plugin.
Step 2: Check the plugin is installed and active
So we need to first check that the Plausible Analytics plugin is activated and then do the redirect.
That’s what we do in the code below at line 4.
// redirect our Dashboard > Home page to a custom url
add_action('admin_init', 'c_admin_redirects');
function c_admin_redirects() {
if ( is_plugin_active('plausible-analytics/plausible-analytics.php') ) {
}
}
Code language: PHP (php)
You will need to change this line to whatever plugin you’re trying to redirect to and find the necessary php file within that plugin to add to the code above.
Let’s do an example together and, in doing so, you’ll be able to do this step yourself.
Another plugin that I will redirect to sometimes is the Just Writing Statistics plugin which shows me how many words I’ve written on my Blog this month and looks something like this:
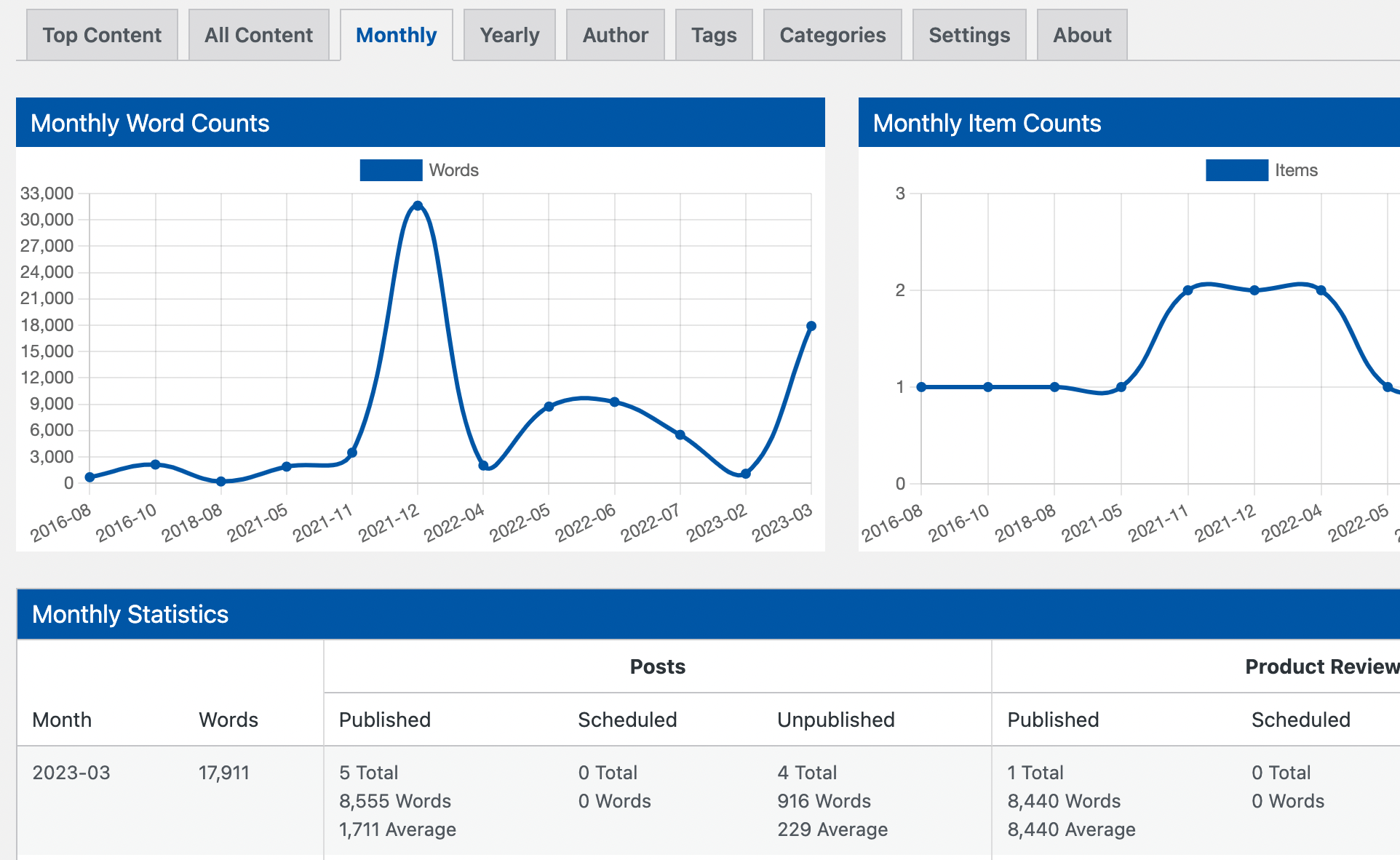
This helps motivate me to keep writing and I like to have it front and center during times I’m producing content.
[bricks_template id=”72291″]If I go to my /wp-content/plugins/ folder and open up this plugin’s files, I can see a file named just-writing-statistics.php
inside (1).
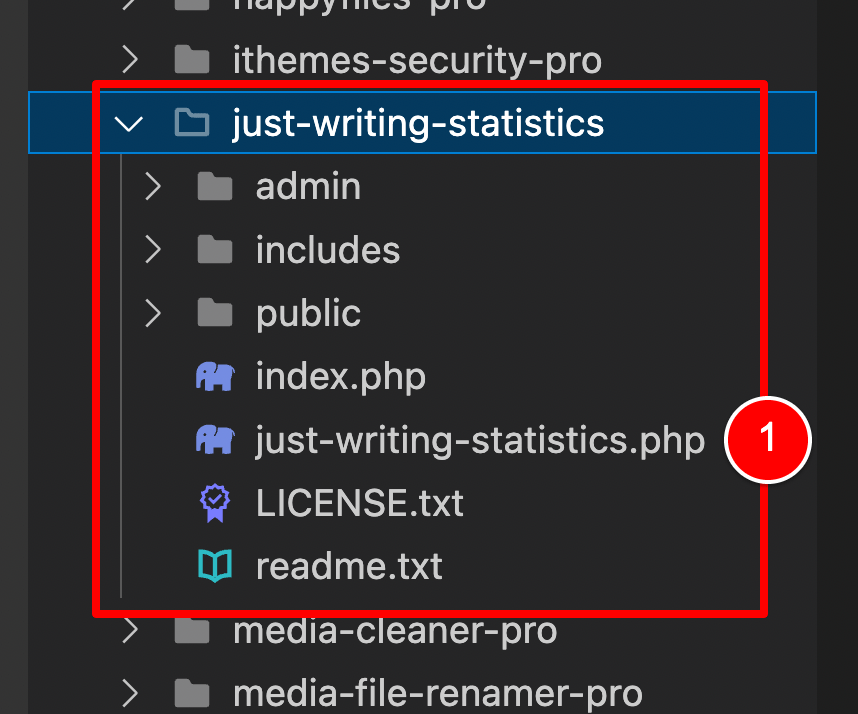
In a lot of plugins, inside a Plugin’s files, you will find a .php file that matches the name of the plugin and this will be the file you want to use.
For example, if I open up the Happy Files plugin I use, I will find happyfiles-pro.php
inside.
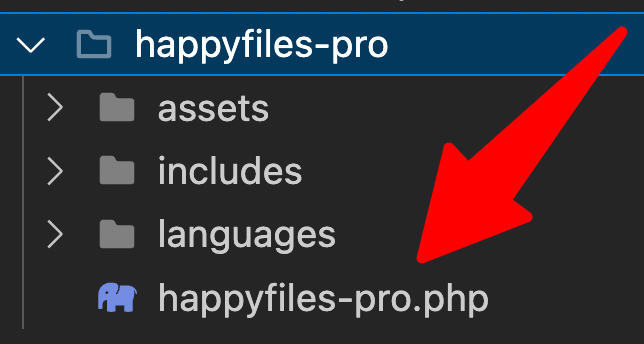
But this won’t always be the case – so you need to be careful here.
If I explore the Advanced Custom Fields Pro plugin’s files, I only see one .php file named acf.php
(1) and NO .php
file called advanced-custom-fields-pro.php
.
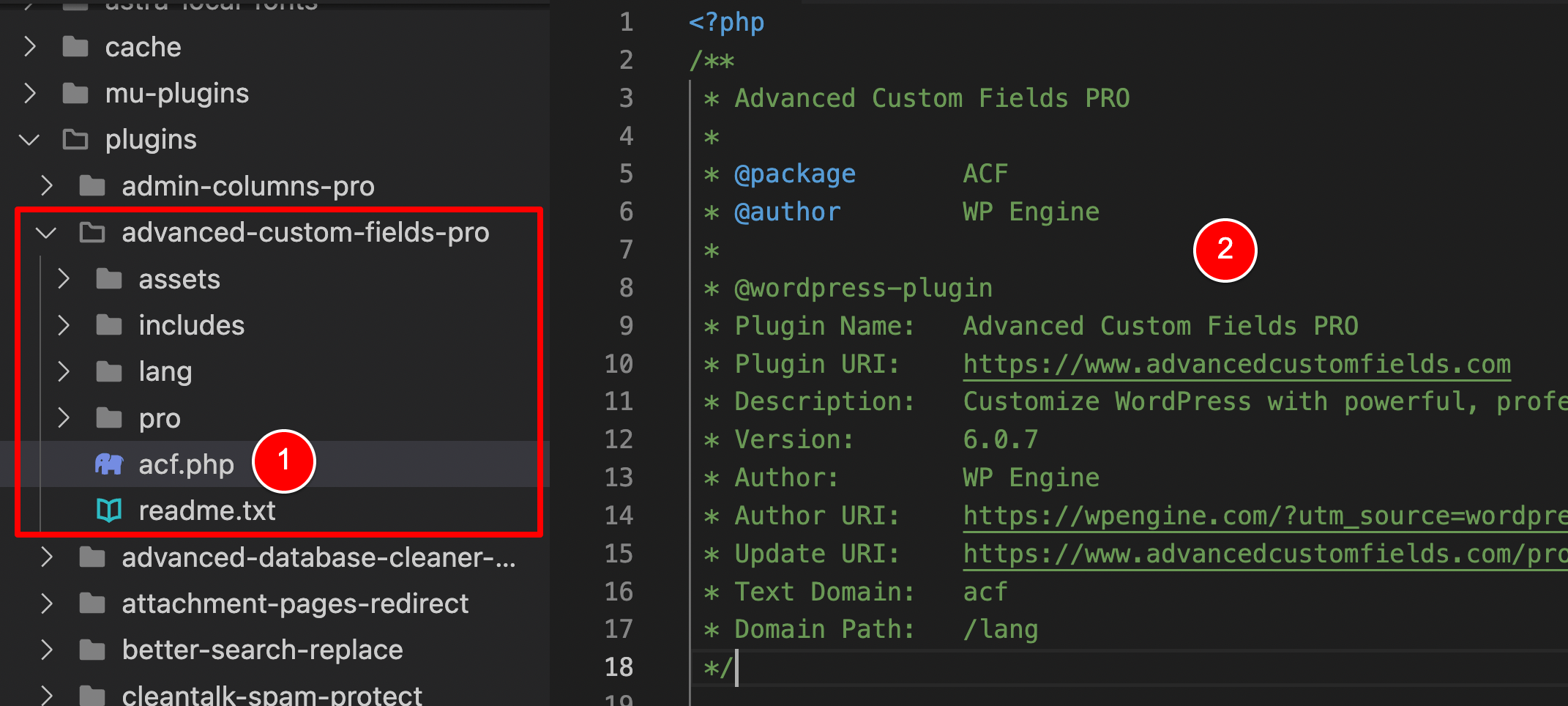
But if I open up that acf.php
file I can see the commented metadata about the Plugin that WordPress uses (and requires) to register a plugin (2).
So for the plugin, you want to redirect to – just look for the PHP file that has this commented metadata at the top of the file and that’s what you’ll use in your redirect function.
But as we saw before – in my case, there is a matching PHP file for the Just Writing Statistics plugin…
So if I want to redirect Dashboard > Home to this plugin, I would update the code to the below.
// redirect our Dashboard > Home page to a custom url
add_action('admin_init', 'c_admin_redirects');
function c_admin_redirects() {
if ( is_plugin_active('just-writing-statistics/just-writing-statistics.php') ) {
}
}
Code language: PHP (php)
Step 3: Check we are on Dashboard > Home using PHP
Now that we have our PHP check in place to ensure we only trigger our redirect if the plugin we’re redirecting to is currently active within WordPress…
The next lines of code we’ll go through are these two here:
// redirect our Dashboard > Home page to a custom url
add_action('admin_init', 'c_admin_redirects');
function c_admin_redirects() {
if ( is_plugin_active('plausible-analytics/plausible-analytics.php') ) {
global $pagenow;
if($pagenow == 'index.php' && !isset($_GET['page'])){
}
}
}
Code language: PHP (php)
The first question you probably have is…
What is
$pagenow
?
To show you, I will add the below line of code temporarily which will output the value of the variable named $pagenow in our admin area.
add_action('admin_init', 'c_admin_redirects');
function c_admin_redirects() {
if ( is_plugin_active('plausible-analytics/plausible-analytics.php') ) {
global $pagenow;
echo 'The pagenow = ' . $pagenow;
}
}
Code language: PHP (php)
With that line of code added, I will go to Dashboard > Home and we can see that $pagenow
has the value of index.php
(1) which matches the part of the URL after …/wp-admin/ (2).
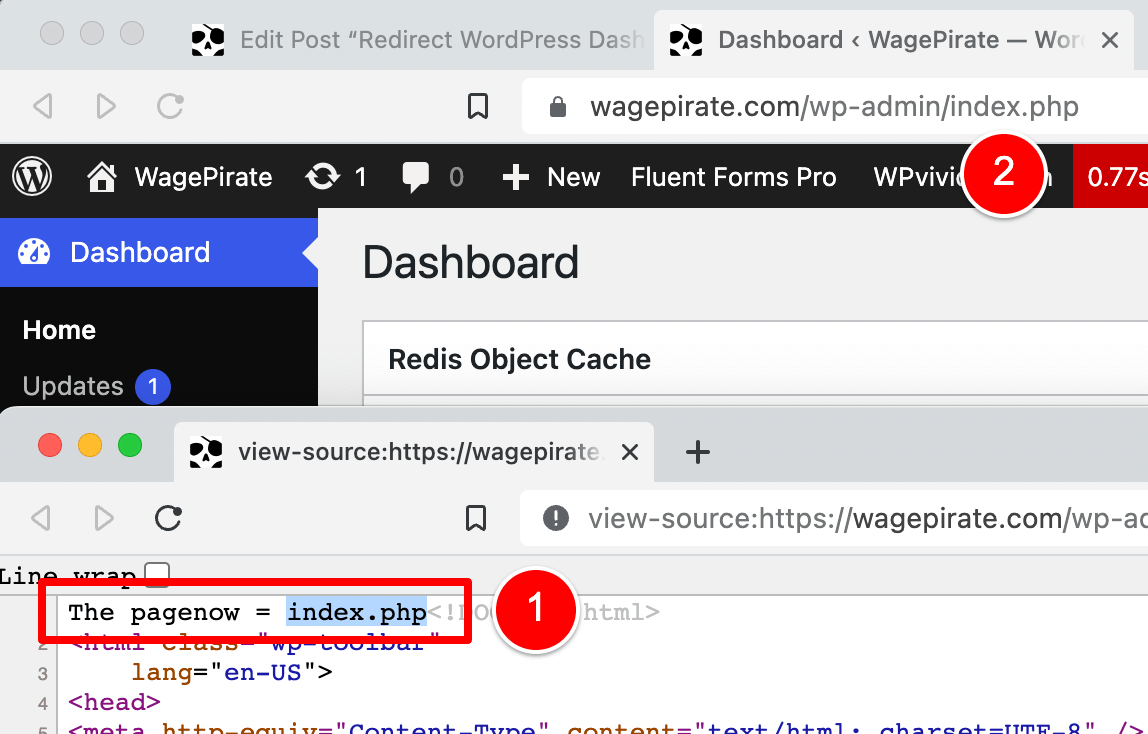
But I’m not showing you this because I enjoy taking screenshots – this means something!
If I go to Dashboard > Analytics (which is the page that has the Plausible Analytics reports) I can see that the $pagenow variable has the SAME value of index.php
that our Dashboard > Home page has.
The URL also has index.php (2) but after this we have ?page=plausible-analytics-statistics
(3)
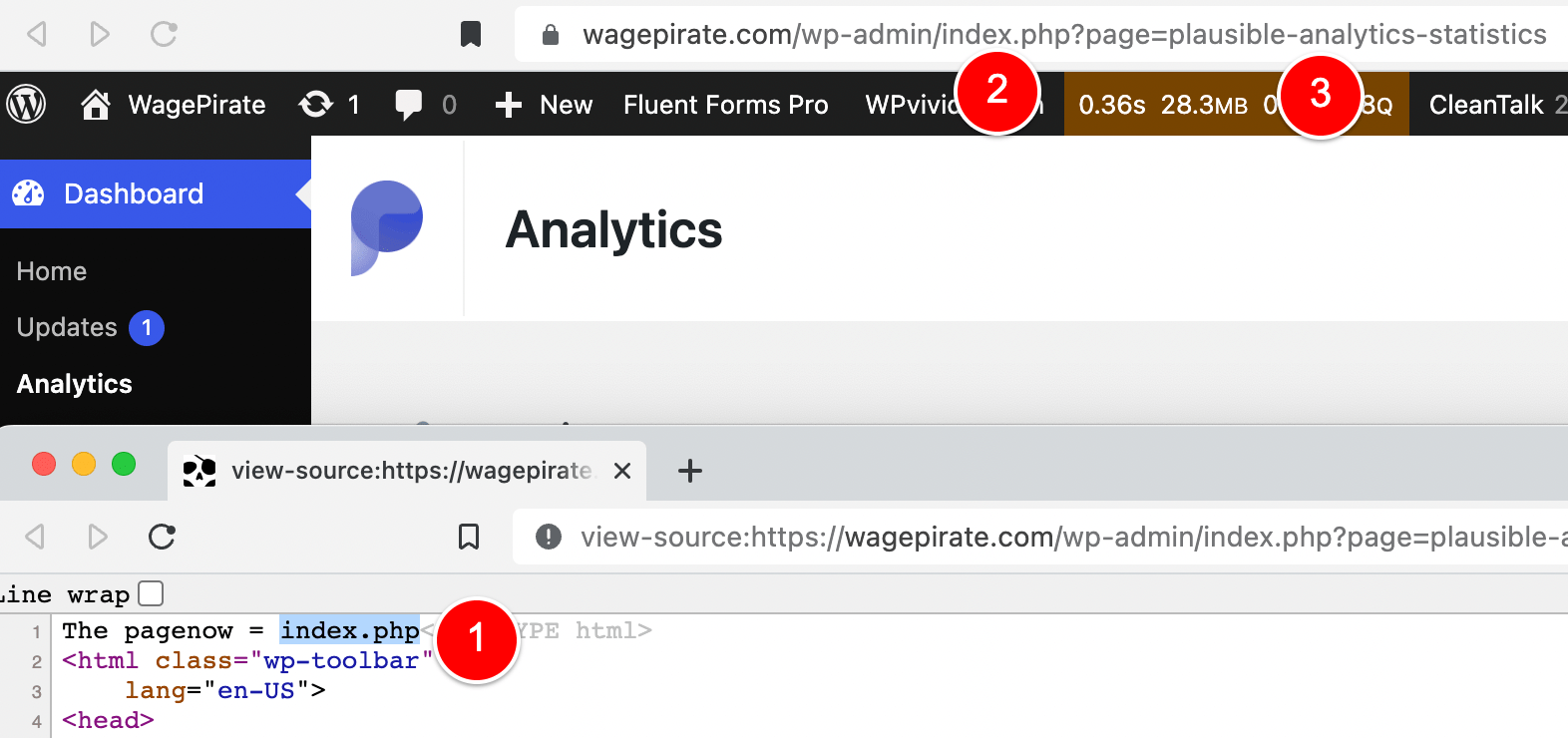
This is why we can’t just check to make sure that we are on the index.ph
p page…
if($pagenow == 'index.php')
Code language: PHP (php)
Because our logic is to redirect from Dashboard > Home to Dashboard > Analytics and both of them are on index.php.
This would cause an infinite redirect and properly crash our website.
So looking at the difference between these two dashboard pages, only 1 of them contains the URL variable ?page=
(the analytics page).
So we can just check to see if that page URL variable exists (i.e. is set).
- If the page URL variable DOES exist:
Then we are NOT accessing the Dashboard > Home page and so we don’t want to redirect and our function will do nothing. - If the page URL variable DOES NOT exist:
Then we are accessing Dashboard > Home and we do want to redirect.
And that’s the exact logic the below condition provides us:
if($pagenow == 'index.php' && !isset($_GET['page']))
Code language: PHP (php)
Step 3: Redirect to our target URL
The next part of our function we’ll review is the actual redirect itself, which is the following 2 lines of code:
if($pagenow == 'index.php' && !$_GET['page']){
wp_redirect(admin_url("admin.php?page=just-writing-statistics", 'https'), 301);
exit;
}
Code language: PHP (php)
Let me show you how to find the URL to put here for your redirect.
Go to the page that you want to redirect Dashboard > Home to and copy the part AFTER https:<yourwebsite.com>/wp-admin/
(it will usually start with admin.php or index.php).
For the Just Writing Statistics plugin I looked at earlier, the part of the URL we want to use is highlighted below.
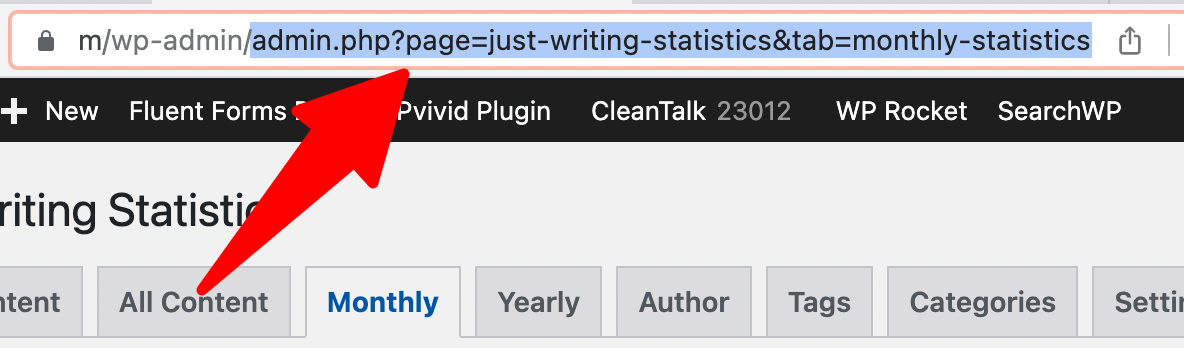
which is…
admin.php?page=just-writing-statistics
So I would paste as per the below:
if($pagenow == 'index.php' && !$_GET['page']){
wp_redirect(admin_url("admin.php?page=just-writing-statistics", 'https'), 301);
exit;
}
Code language: PHP (php)
If I were wanting to redirect to Plausible Analytics, I would copy index.php?page=plausible-analytics-statistics
…
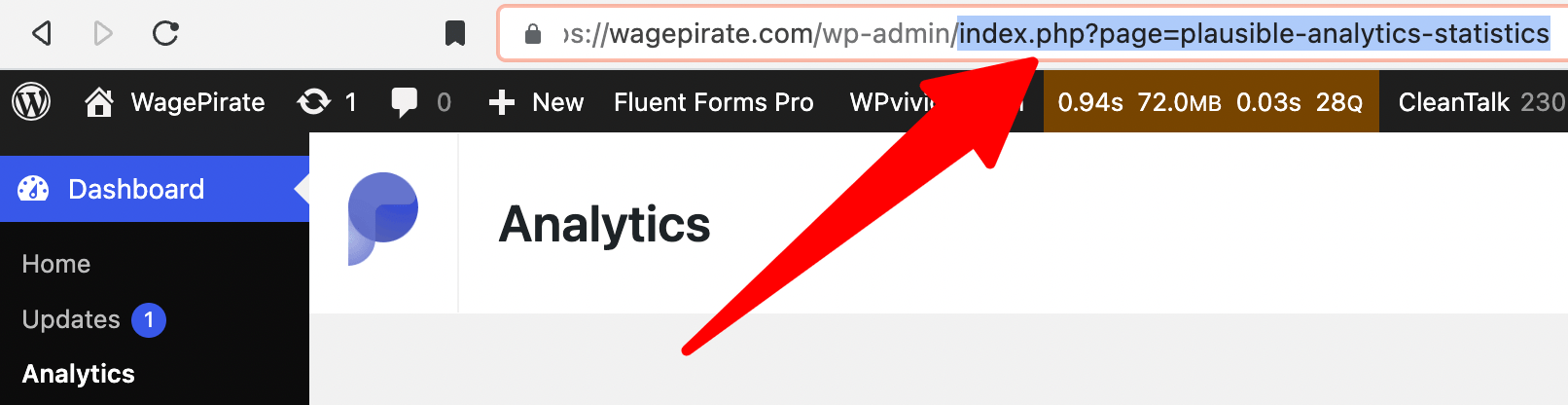
and the code would be as per below.
if($pagenow == 'index.php' && !$_GET['page']){
wp_redirect(admin_url("index.php?page=plausible-analytics-statistics", 'https'), 301);
exit;
}
Code language: PHP (php)
Step 4: Exit
Worth noting is that directly after we trigger our wp_redirect();
function we do the following:
exit;
Code language: PHP (php)
exit;
terminates the script and stops any subsequent code on the page from running.
If we are redirecting to another page, we don’t want to waste any more time trying to load the page we are leaving – thus, we exit (aka stop) rendering the page.
What next?
And will all that information, you should be well on your way to redirecting from Dashboard > Home to a page that better suits you, your business and your website goals.
Let me know in the comments below what plugin page you’re redirecting to on your website with the code from today’s article…
And why.
I’d love to know!
Leave a Reply